Google
Maps with Autocomplete and Location Enable/Disable
In
this blog, I will show you how to integrate google map into our
application and display maps depending on enabling and disabling of
location on your mobile/system. Also change the location dynamically
when user enters a location in google autocomplete text box.
1)Add
Google API Key as shown below:
<script src="https://maps.googleapis.com/maps/api/js?libraries=places&key=API KEY"></script>
2) Add angular directive(googleplace) for autocomplete in App.js:
2) Add angular directive(googleplace) for autocomplete in App.js:
MY_APP.directive('googleplace', function() {
return {
require : 'ngModel',
link : function(scope, element, attrs, model) {
var options = {
types : []
};
scope.gPlace = new google.maps.places.Autocomplete(element[0],
options);
google.maps.event.addListener(scope.gPlace, 'place_changed',
function() {
scope.$apply(function() {
model.$setViewValue(element.val());
});
});
}
};
});
3) HTML code for displaying map:
<div class="container-fluid" ng-controller="MapController">
<form id="form1" ng-init="currentlocation()">
<div>
<input id="address" type="text" class="form-control" ng-model="address" value="address" googleplace >
<input id="submit" class="btn btn-success" type="button" value="Show" >
</div>
<div id="map_canvas" style="width: 1335px; height:635px;"></div>
</form></div>
4)Controller.js code for displaying the map:
i) When location is enabled below code is executed along with text box to change the location:
$scope.currentlocation = function () {
var myOptions = {
center: {lat: -35.397, lng: 151.644},
zoom: 6,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(document.getElementById("map_canvas"), myOptions);
var geocoder = new google.maps.Geocoder();
document.getElementById('submit').addEventListener('click', function () {
geocodeAddress(geocoder, map);
});
infoWindow = new google.maps.InfoWindow;
// Try HTML5 geolocation.
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(function (position) {
var pos = {
lat: position.coords.latitude,
lng: position.coords.longitude
};
var marker = new google.maps.Marker({
position: pos,
map: map
});
infoWindow.setPosition(pos);
map.setCenter(pos);
}, function () {
handleLocationError(true, infoWindow, map.getCenter());
});
} else {
// Browser doesn't support Geolocation
handleLocationError(false, infoWindow, map.getCenter());
}
};
function handleLocationError(browserHasGeolocation, infoWindow, pos) {
infoWindow.setPosition(pos);
$scope.initMap1();
infoWindow.setContent(browserHasGeolocation ?
'Error: The Geolocation service failed.' :
'Error: Your browser doesn\'t support geolocation.');
infoWindow.open(map);
};
ii) When location is disabled below code is executed along with text box:
$scope.initMap1 = function () {
var uluru = {lat: 17.811265, lng: 83.389743};
var map = new google.maps.Map(document.getElementById('map_canvas'), {
zoom: 12,
center: uluru
});
var marker = new google.maps.Marker({
position: uluru,
map: map
});
var geocoder = new google.maps.Geocoder();
document.getElementById('submit').addEventListener('click', function () {
geocodeAddress(geocoder, map);
});
};
function geocodeAddress(geocoder, resultsMap) {
var address = document.getElementById('address').value;
geocoder.geocode({'address': address}, function (results, status) {
if (status === 'OK') {
resultsMap.setCenter(results[0].geometry.location);
var marker = new google.maps.Marker({
map: resultsMap,
position: results[0].geometry.location
});
} else {
alert('Geocode was not successful for the following reason: ' + status);
}
});
};
5)Below are the screenshots for displaying maps :
i)When user enables location by clicking on allow in mobile/system:
ii)Enter desired location in google autocomplete text box to display a different location and click on save as shown below: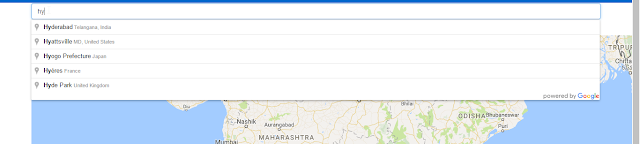
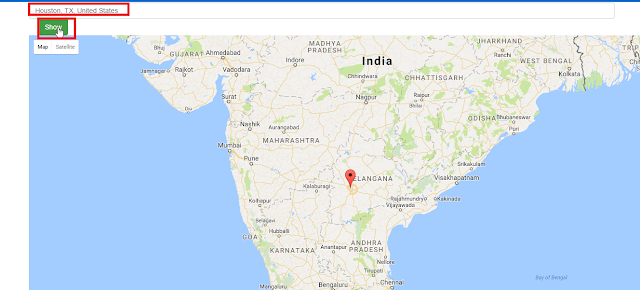
iv)User entered location will be displayed on map as shown below:
v)When user disables his location ,static location as given in code will be displayed as shown below along with a text box:
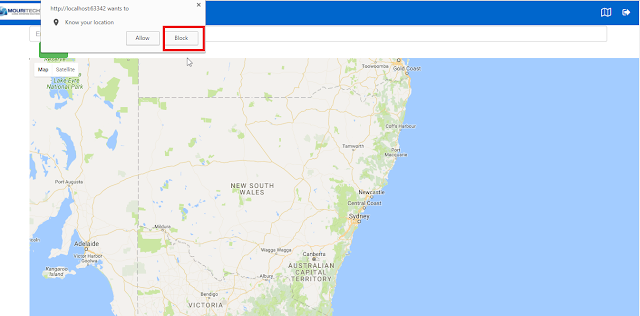
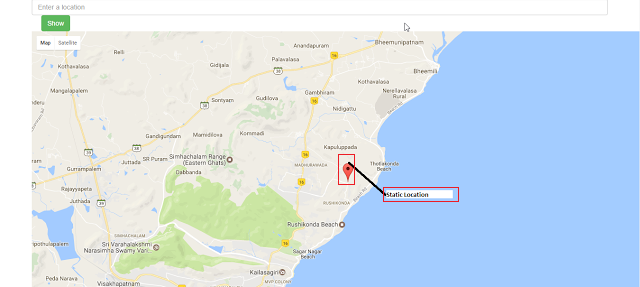
vi)Enter desired location in google autocomplete text box to display a different location and click on save as shown below:
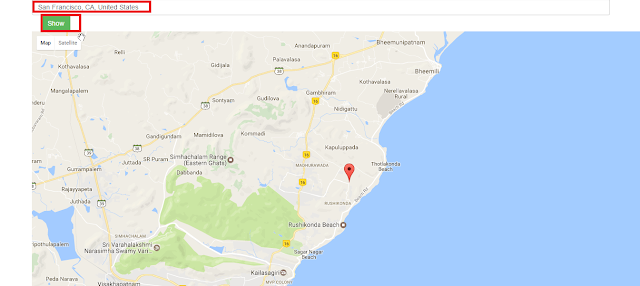
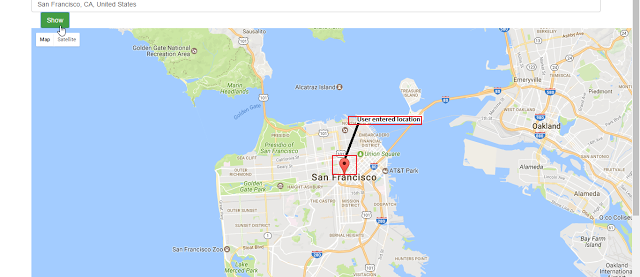
This is how we integrate google autocomplete along with enable/disable functionalites to a Google map in the application.
Thanks,
Lakshmi Alekhya Vaddi
alekhya4c4@gmail.com
MOURITECH PVT LTD.
www.mouritech.com
Comments
Post a Comment